Javax Speech Download
Overview Package Class Tree Index Help | ||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |
SUMMARY: INNER FIELD CONSTR METHOD | DETAIL: FIELD CONSTR METHOD |
Interface javax.speech.synthesis.Synthesizer
- public abstract interface Synthesizer
- extends Engine
A link from the Desktop Java Java Speech API leads to the SourceForge page for FreeTTS. The FAQ says: The Java Speech API (JSAPI) is not part of the JDK and Sun does not ship an implementation of JSAPI. Instead, we work with third party speech companies to encourage the availability of multiple implementations. Just ONE TINY, LITTLE problem. Javax.speech is within the IBM file I downloaded, I uncompressed it, and have copied and pasted it to a different directory, which is in the CLASSPATH variable, HOWEVER, the program I've got which uses the javax.speech package, when I compile it, it says 'package javax.speech does not exist'.
TheSynthesizer
interface provides primary access to speech synthesis capabilities. The Synthesizer
interface extends the Engine
interface. Thus, any Synthesizer
implements basic speech engine capabilities plus the specialized capabilities required for speech synthesis. The primary functions provided by the Synthesizer
interface are the ability to speak text, speak Java Speech Markup Language text, and control an output queue of objects to be spoken.
Creating a Synthesizer
Typically, a Synthesizer is created by a call to the Central.createSynthesizer
method. The procedures for locating, selecting, creating and initializing a Synthesizer
are described in the documentation for Central
class.
Synthesis Package: Inherited and Extended Capabilities
A synthesis
package inherits many of its important capabilities from the Engine
interface and its related support classes and interfaces. The synthesis
package adds specialized functionality for performing speech synthesis.
- Inherits location mechanism by
Central.availableSynthesizers
method andEngineModeDesc
. - Extends
EngineModeDesc
asSynthesizerModeDesc
. - Inherits
allocate
anddeallocate
methods from theEngine
interface. - Inherits
pause
andresume
methods from theEngine
interface. - Inherits
getEngineState
,waitEngineState
andtestEngineState
methods from theEngine
interface. - Inherits the
DEALLOCATED
,ALLOCATED
,ALLOCATING_RESOURCES
,DEALLOCATING_RESOURCES
,PAUSED
andRESUMED
states from theEngine
interface. - Adds
QUEUE_EMPTY
andQUEUE_NOT_EMPTY
sub-states to theALLOCATED
state. - Inherits audio management: see
Engine.getAudioManager
andAudioManager
. - Inherits vocabulary management: see Engine.getVocabManager and VocabManager.
- Inherits
addEngineListener
andremoveEngineListener
methods and uses theEngineListener
interface. Extends EngineListener
interface toSynthesizerListener
.- Adds
speak(Speakable, Listener)
,speak(URL, Listener)
,speak(String, Listener)
andspeakPlainText(String)
methods to place text on the output queue of the synthesizer. - Adds
phoneme(String)
method that converts text to phonemes. - Adds
enumerateQueue
,cancel()
,cancel(Object)
andcancelAll
methods for management of output queue.
Speaking Text
The basic function of a Synthesizer
is to speak text provided to it by an application. This text can be plain Unicode text in a String
or can be marked up using the Java Speech Markup Language (JSML).
Plain text is spoken using the speakPlainText
method. JSML text is spoken using one of the three speak
methods. The speak
methods obtain the JSML text for a Speakable
object, from a URL
, or from a String
.
[Note: JSML text provided programmatically (by a Speakable
object or a String
) does not require the full XML header. JSML text obtained from a URL requires the full XML header.]
A synthesizer is mono-lingual (it speaks a single language) so the text should contain only the single language of the synthesizer. An application requiring output of more than one language needs to create multiple Synthesizer
object through Central
. The language of the Synthesizer
should be selected at the time at which it is created. The language for a created Synthesizer
can be checked through the Locale
of its EngineModeDesc
(see getEngineModeDesc
).
Each object provided to a synthesizer is spoken independently. Sentences, phrases and other structures should not span multiple call to the speak
methods.
Synthesizer State System
Synthesizer
extends the state system of the generic Engine
interface. It inherits the four basic allocation states, plus the PAUSED
and RESUMED
states.
Synthesizer
adds a pair of sub-states to the ALLOCATED
state to represent the state of the speech output queue (queuing is described in more detail below). For an ALLOCATED
Synthesizer
, the speech output queue is either empty or not empty: represented by the states QUEUE_EMPTY
and QUEUE_NOT_EMPTY
.
The queue status is independent of the pause/resume status. Pausing or resuming a synthesizer does not effect the queue. Adding or removing objects from the queue does not effect the pause/resume status. The only form of interaction between these state systems is that the Synthesizer
only speaks in the RESUMED
state, and therefore, a transition from QUEUE_NOT_EMPTY
to QUEUE_EMPTY
because of completion of speaking an object is only possible in the RESUMED
state. (A transition from QUEUE_NOT_EMPTY
to QUEUE_EMPTY
is possible in the PAUSED
state only through a call to one of the cancel
methods.)
Speech Output Queue
A synthesizer implements a queue of items provided to it through the speak
and speakPlainText
methods. The queue is 'first-in, first-out (FIFO)' -- the objects are spoken in exactly he order in which they are received. The object at the top of the queue is the object that is currently being spoken or about to be spoken.
The QUEUE_EMPTY
and QUEUE_NOT_EMPTY
states of a Synthesizer
indicate the current state of of the speech output queue. The state handling methods inherited from the Engine
interface (getEngineState
, waitEngineState
and testEngineState
) can be used to test the queue state.
The items on the queue can be checked with the enumerateQueue
method which returns a snapshot of the queue.
The cancel
methods allows an application to (a) stop the output of item currently at the top of the speaking queue, (b) remove an arbitrary item from the queue, or (c) remove all items from the output queue.
Applications requiring more complex queuing mechanisms (e.g. a prioritized queue) can implement their own queuing objects that control the synthesizer.
Pause and Resume
The pause and resume methods (inherited from the javax.speech.Engine
interface) have behavior like a 'tape player'. Pause stops audio output as soon as possible. Resume restarts audio output from the point of the pause. Pause and resume may occur within words, phrases or unnatural points in the speech output.
Pause and resume do not affect the speech output queue.
In addition to the ENGINE_PAUSED
and ENGINE_RESUMED
events issued to the EngineListener
(or SynthesizerListener
), SPEAKABLE_PAUSED
and SPEAKABLE_RESUMED
events are issued to appropriate SpeakableListeners
for the Speakable
object at the top of the speaking queue. (The SpeakableEvent
is first issued to any SpeakableListener
provided with the speak
method, then to each SpeakableListener
attached to the Synthesizer
. Finally, the EngineEvent
is issued to each SynthesizerListener
and EngineListener
attached to the Synthesizer
.)
Applications can determine the approximate point at which a pause occurs by monitoring the WORD_STARTED
events.
- See Also:
- Central, Speakable, SpeakableListener, EngineListener, SynthesizerListener
Field Summary | |
static long | QUEUE_EMPTY Bit of state that is set when the speech output queue of a Synthesizer is empty. |
static long | QUEUE_NOT_EMPTY Bit of state that is set when the speech output queue of a Synthesizer is not empty. |
Method Summary | |
void | addSpeakableListener(SpeakableListener listener) Request notifications of all SpeakableEvents for all speech output objects for this Synthesizer . |
void | cancelAll() Cancel all objects in the synthesizer speech output queue and stop speaking the current top-of-queue object. |
void | cancel() Cancel output of the current object at the top of the output queue. |
void | cancel(Object source) Remove a specified item from the speech output queue. |
Enumeration | enumerateQueue() Return an Enumeration containing a snapshot of all the objects currently on the speech output queue. |
SynthesizerProperties | getSynthesizerProperties() Return the SynthesizerProperties object (a JavaBean). |
String | phoneme(String text) Returns the phoneme string for a text string. |
void | removeSpeakableListener(SpeakableListener listener) Remove a SpeakableListener from this Synthesizer . |
void | speakPlainText(String text, SpeakableListener listener) Speak a plain text string. |
void | speak(Speakable JSMLtext, SpeakableListener listener) Speak an object that implements the Speakable interface and provides text marked with the Java Speech Markup Language. |
void | speak(URL JSMLurl, SpeakableListener listener) Speak text from a URL formatted with the Java Speech Markup Language. |
void | speak(String JSMLText, SpeakableListener listener) Speak a string containing text formatted with the Java Speech Markup Language. |
Field Detail |
QUEUE_EMPTY
Bit of state that is set when the speech output queue of aSynthesizer
is empty. The QUEUE_EMPTY
state is a sub-state of the ALLOCATED
state. An allocated Synthesizer
is always in either the QUEUE_NOT_EMPTY
or QUEUE_EMPTY
state. A Synthesizer
is always allocated in the QUEUE_EMPTY
state. The Synthesizer
transitions from the QUEUE_EMPTY
state to the QUEUE_NOT_EMPTY
state when a call to one of the speak
methods places an object on the speech output queue. A QUEUE_UPDATED
event is issued to indicate this change in state.
A Synthesizer
returns from the QUEUE_NOT_EMPTY
state to the QUEUE_EMPTY
state once the queue is emptied because of completion of speaking all objects or because of a cancel
.
The queue status can be tested with the waitQueueEmpty
, getEngineState
and testEngineState
methods. To block a thread until the queue is empty:
- See Also:
- QUEUE_NOT_EMPTY, ALLOCATED, getEngineState, waitEngineState, testEngineState, QUEUE_UPDATED
QUEUE_NOT_EMPTY
Bit of state that is set when the speech output queue of aSynthesizer
is not empty. The QUEUE_NOT_EMPTY
state is a sub-state of the ALLOCATED
state. An allocated Synthesizer
is always in either the QUEUE_NOT_EMPTY
or QUEUE_EMPTY
state. A Synthesizer
enters the QUEUE_NOT_EMPTY
from the QUEUE_EMPTY
state when one of the speak
methods is called to place an object on the speech output queue. A QUEUE_UPDATED
event is issued to mark this change in state.
A Synthesizer
returns from the QUEUE_NOT_EMPTY
state to the QUEUE_EMPTY
state once the queue is emptied because of completion of speaking all objects or because of a cancel
.
- See Also:
- QUEUE_EMPTY, ALLOCATED, getEngineState, waitEngineState, testEngineState, QUEUE_UPDATED
Method Detail |
speak
Speak an object that implements theSpeakable
interface and provides text marked with the Java Speech Markup Language. The Speakable
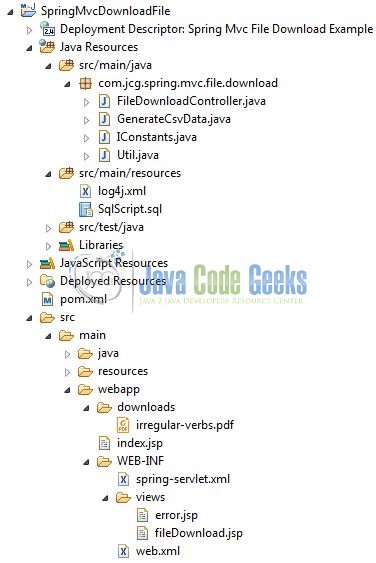
RESUMED
Javax Jar File Download
state. The synthesizer first requests the text of the Speakable
by calling its getJSMLText
method. It then checks the syntax of the JSML markup and throws a JSMLException
if any problems are found. If the JSML text is legal, the text is placed on the speech output queue.
When the speech output queue is updated, a QUEUE_UPDATE
event is issued to SynthesizerListeners
.
Events associated with the Speakable
object are issued to the SpeakableListener
object. The listener may be null
. A listener attached with this method cannot be removed with a subsequent remove call. The source for the SpeakableEvents
is the JSMLtext
object.
SpeakableEvents
can also be received by attaching a SpeakableListener
to the Synthesizer
with the addSpeakableListener
method. A SpeakableListener
attached to the Synthesizer
receives all SpeakableEvents
for all speech output items of the synthesizer (rather than for a single Speakable
).
The speak call is asynchronous: it returns once the text for the Speakable
has been obtained, checked for syntax, and placed on the synthesizer's speech output queue. An application needing to know when the Speakable
has been spoken should wait for the SPEAKABLE_ENDED
event to be issued to the SpeakableListener
object. The getEngineState
, waitEngineState
and enumerateQueue
methods can be used to determine the speech output queue status.
An object placed on the speech output queue can be removed with one of the cancel
methods.
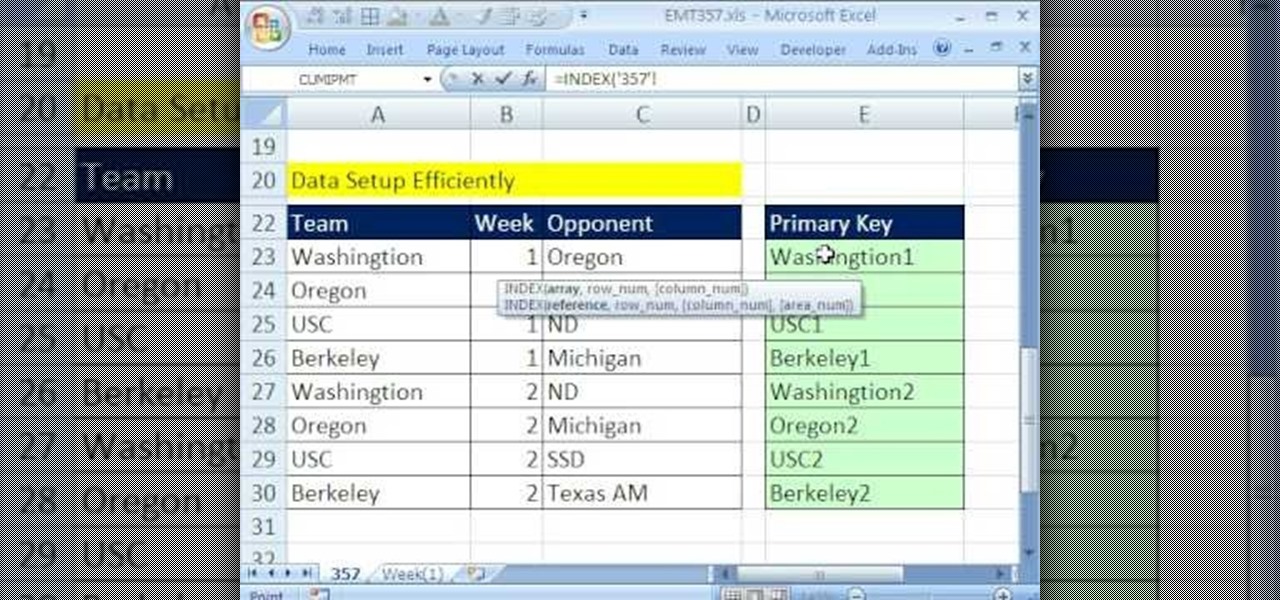
The speak
methods operate as defined only when a Synthesizer
is in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
JSMLText
- object implementing the Speakable interface that provides Java Speech Markup Language text to be spokenlistener
- receives notification of events as synthesis output proceeds- Throws:
- JSMLException - if any syntax errors are encountered in
JSMLtext
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- speak(String, SpeakableListener), speak(URL, SpeakableListener), speakPlainText(String, SpeakableListener), SpeakableEvent, addSpeakableListener
speak
Speak text from a URL formatted with the Java Speech Markup Language. The text is obtained from the URL, checked for legal JSML formatting, and placed at the end of the speaking queue. It is spoken once it reaches the top of the queue and the synthesizer is in the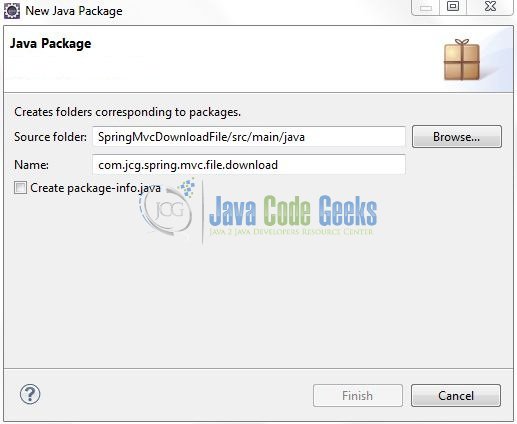
RESUMED
state. In other respects is it identical to the speak
method that accepts a Speakable
object. The source of a SpeakableEvent
issued to the SpeakableListener
is the URL
.
Because of the need to check JSML syntax, this speak
method returns only once the complete URL is loaded, or until a syntax error is detected in the URL stream. Network delays will cause the method to return slowly.
Note: the full XML header is required in the JSML text provided in the URL. The header is optional on programmatically generated JSML (ie. with the speak(String, Listener)
and speak(Speakable, Listener)
methods.
The speak
methods operate as defined only when a Synthesizer
is in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
JSMLurl
- URL containing Java Speech Markup Language text to be spokenJSMLException
- if any syntax errors are encountered inJSMLtext
listener
- receives notification of events as synthesis output proceeds- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- speak(Speakable, SpeakableListener), speak(String, SpeakableListener), speakPlainText(String, SpeakableListener), SpeakableEvent, addSpeakableListener
speak
Speak a string containing text formatted with the Java Speech Markup Language. The JSML text is checked for formatting errors and aJSMLException
is thrown if any are found. If legal, the text is placed at the end of the speaking queue and will be spoken once it reaches the top of the queue and the synthesizer is in the RESUMED
state. In all other respects is it identical to the speak
method that accepts a Speakable
object. Javax.speech.recognition Package Download
The source of a SpeakableEvent
issued to the SpeakableListener
is the String
.
The speak
methods operate as defined only when a Synthesizer
is in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
JSMLText
- String contains Java Speech Markup Language text to be spokenlistener
- receives notification of events as synthesis output proceedsJSMLException
- if any syntax errors are encountered inJSMLtext
- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- speak(Speakable, SpeakableListener), speak(URL, SpeakableListener), speakPlainText(String, SpeakableListener)
speakPlainText
Speak a plain text string. The text is not interpreted as containing the Java Speech Markup Language so JSML elements are ignored. The text is placed at the end of the speaking queue and will be spoken once it reaches the top of the queue and the synthesizer is in theRESUMED
state. In other respects it is similar to the speak
method that accepts a Speakable
object. The source of a SpeakableEvent
issued to the SpeakableListener
is the String
object.
The speak
methods operate as defined only when a Synthesizer
is in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
JSMLText
- String contains plaing text to be spokenlistener
- receives notification of events as synthesis output proceeds- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- speak(Speakable, SpeakableListener), speak(URL, SpeakableListener), speak(String, SpeakableListener)
phoneme
Returns the phoneme string for a text string. The return string uses the International Phonetic Alphabet subset of Unicode. The input string is expected to be simple text (for example, a word or phrase in English). The text is not expected to contain punctuation or JSML markup. If the Synthesizer
does not support text-to-phoneme conversion or cannot process the input text it will return null
.
If the text has multiple pronunciations, there is no way to indicate which pronunciation is preferred.
The phoneme
method operate as defined only when a Synthesizer
is in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
text
- plain text to be converted to phonemes- Returns:
- phonemic representation of text or
null
- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states
enumerateQueue
Return anEnumeration
containing a snapshot of all the objects currently on the speech output queue. The first item is the top of the queue. An empty queue returns a null object. If the return value is non-null then each object it contains is guaranteed to be a SynthesizerQueueItem
object representing the source object (Speakable
object, URL
, or a String
) and the JSML or plain text obtained from that object.
A QUEUE_UPDATED
event is issued to each SynthesizerListener
whenever the speech output queue changes. A QUEUE_EMPTIED
event is issued whenever the queue the emptied.
This method returns only the items on the speech queue placed there by the current application or applet. For security reasons, it is not possible to inspect items placed by other applications.
The items on the speech queue cannot be modified by changing the object returned from this method.
The enumerateQueue
method works in the ALLOCATED
state. The call blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and completes when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
Wifislax iso. Aug 9, 2017 - Booting into the KDE environment, with persistent mode enabled to save your session, seams to be the best choice for the new Wifislax user. It provides a familiar user interface, with popular open source applications, such as the Mozilla Firefox web browser, ISO Master, XMMS audio player, and SMPlayer.
- Returns:
- an
Enumeration
of the speech output queue or null - Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- SynthesizerQueueItem, QUEUE_UPDATED, QUEUE_EMPTIED, addEngineListener
cancel
Cancel output of the current object at the top of the output queue. ASPEAKABLE_CANCELLED
event is issued to appropriate SpeakableListeners
. If there is another object in the speaking queue, it is moved to top of queue and receives the TOP_OF_QUEUE
event. If the Synthesizer
is not paused, speech output continues with that object. To prevent speech output continuing with the next object in the queue, call pause
before calling cancel
.
A SynthesizerEvent
is issued to indicate QUEUE_UPDATED
(if objects remain on the queue) or QUEUE_EMPTIED
(if the cancel leaves the queue empty).
It is not an exception to call cancel if the speech output queue is empty.
The cancel
methods work in the ALLOCATED
state. The calls blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and complete when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- cancel(Object), cancelAll(), QUEUE_UPDATED, QUEUE_EMPTIED, TOP_OF_QUEUE, SPEAKABLE_CANCELLED
cancel
Remove a specified item from the speech output queue. The source object must be one of the items passed to aspeak
method. A SPEAKABLE_CANCELLED
event is issued to appropriate SpeakableListeners
. If the source object is the top item in the queue, the behavior is the same as the cancel()
method.
If the source object is not at the top of the queue, it is removed from the queue without affecting the current top-of-queue speech output. A QUEUE_UPDATED
is then issued to SynthesizerListeners
.
If the source object appears multiple times in the queue, only the first instance is cancelled.
Warning: cancelling an object just after the synthesizer has completed speaking it and has removed the object from the queue will cause an exception. In this instance, the exception can be ignored.
The cancel
methods work in the ALLOCATED
state. The calls blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and complete when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Parameters:
source
- object to be removed from the speech output queue- Throws:
- IllegalArgumentException - if the source object is not found in the speech output queue.
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- cancel(), cancelAll(), QUEUE_UPDATED, QUEUE_EMPTIED, SPEAKABLE_CANCELLED
cancelAll
Cancel all objects in the synthesizer speech output queue and stop speaking the current top-of-queue object. The SpeakableListeners
of each cancelled item on the queue receive a SPEAKABLE_CANCELLED
event. A QUEUE_EMPTIED
event is issued to attached SynthesizerListeners
.
A cancelAll
is implictly performed before a Synthesizer
is deallocated.
The cancel
methods work in the ALLOCATED
state. The calls blocks if the Synthesizer
in the ALLOCATING_RESOURCES
state and complete when the engine reaches the ALLOCATED
state. An error is thrown for synthesizers in the DEALLOCATED
or DEALLOCATING_RESOURCES
states.
- Throws:
- EngineStateError - if called for a synthesizer in the
DEALLOCATED
orDEALLOCATING_RESOURCES
states - See Also:
- cancel(), cancel(Object), QUEUE_EMPTIED, SPEAKABLE_CANCELLED
getSynthesizerProperties
Return theSynthesizerProperties
object (a JavaBean). The method returns exactly the same object as the getEngineProperties
method in the Engine
interface. However, with the getSynthesizerProperties
method, an application does not need to cast the return value. The SynthesizerProperties
are available in any state of an Engine
. However, changes only take effect once an engine reaches the ALLOCATED
state.
- Returns:
- the
SynthesizerProperties
object for this engine - See Also:
- getEngineProperties
addSpeakableListener
Request notifications of allSpeakableEvents
for all speech output objects for this Synthesizer
. An application can attach multiple SpeakableListeners
to a Synthesizer
. A single listener can be attached to multiple synthesizers. When an event effects more than one item in the speech output queue (e.g. cancelAll
), the SpeakableEvents
are issued in the order of the items in the queue starting with the top of the queue.
A SpeakableListener
can also provided for an indivudal speech output item by providing it as a parameter to one of the speak
or speakPlainText
methods.
A SpeakableListener
can be attached or removed in any Engine
state.
- Parameters:
listener
- the listener that will receiveSpeakableEvents
- See Also:
- removeSpeakableListener
removeSpeakableListener
Remove aSpeakableListener
from this Synthesizer
. A SpeakableListener
can be attached or removed in any Engine
state.
- See Also:
- addSpeakableListener
Overview Package Class Tree Index Help | ||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |
SUMMARY: INNER FIELD CONSTR METHOD | DETAIL: FIELD CONSTR METHOD |
JavaTM Speech API
Copyright 1997-1998 Sun Microsystems, Inc. All rights reserved
Send comments to javaspeech-comments@sun.com
Now a days the need and the use of the speech synthesizer is increasing everyday and there are lots and lots of new innovations are going on in this field of synthesizer.
The synthesizer is the one which will convert your text input to the speech and for that we’ll be using the Speech Engine that will help us to fulfill our need.
1:
To use the functionality of this you first require to use the Freetts which will provide the functionality of Text to Speech
FreeTTS is a speech synthesis engine written entirely in the Java programming language. FreeTTS was written by the Sun Microsystems Laboratories Speech Team and is based on CMU’s Flite engine. FreeTTS also includes a partial JSAPI 1.0You can download this with the single click here.
Once you have downloaded that .zip then all you need to do is to extract it and have a look inside the folder and move in side the lib. folder “freetts-1.2.2-binfreetts-1.2lib” here you will see collection of .jar files and also the setup for the jsapi.jar file double click on that and that will generate the jsapi.jar file.
Once you have generated the jsapi.jar file now you need to set all this .jar files to the CLASSPATH so that you can import it without any kinda errors.
Else if you are using the Eclipse IDE for your Java Coding than it will be lot easier for you to add all the .jar files to you program by just following step:-
1:- Right click on the new/existing project.
2:-Go to the properties and java Build path — Libraries — add external Jars–select and ok.
else download the .doc that i have used to display the actual way to import it. click here -> Example to import
3:- after importing write the Code
This following code that i have developed through which you can create your speech synthesizer in java.